Last week I released edbee to Github.
Edbee in the essence is an editor QWidget designed for use with Qt.
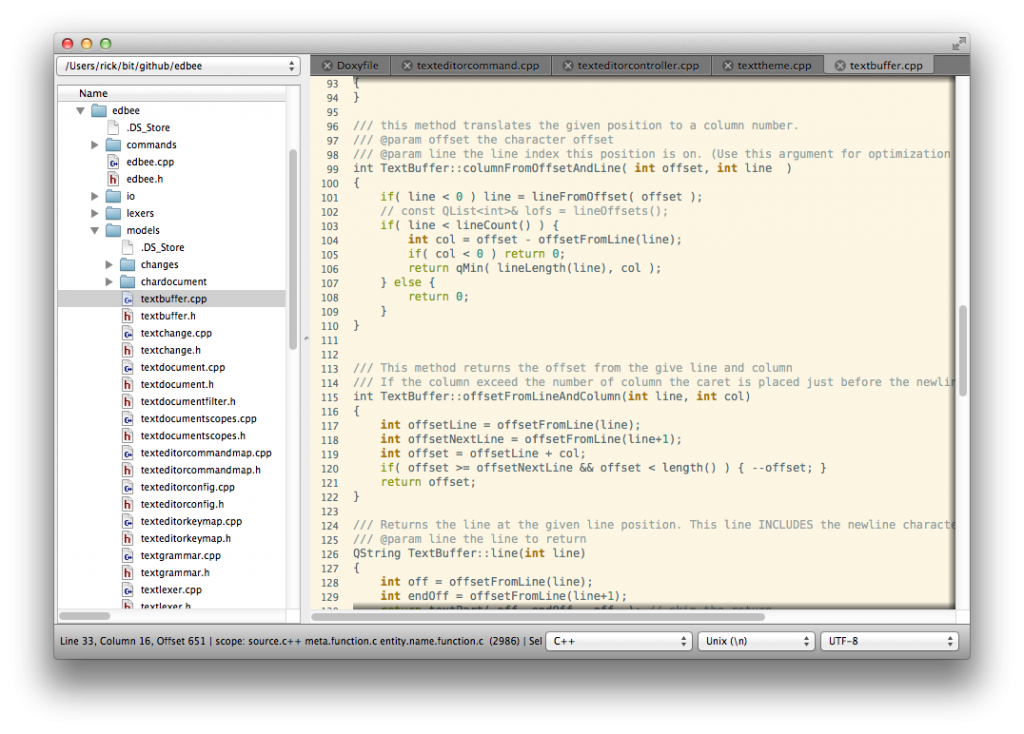
Some history
I've started with this editor component in the summer of 2012 as an in house product.
Edbee has been created because I needed a source-code editor for my Qt Application. I'm developing a very specialistic developers tool, which requires some advanced editor handling. Unfortunately my attempts with diverse standard products failed.
QTextEdit didn't fit my needs, because it was to complex at some points (The richtext part) and way to limited at other parts (replacing the document backend).
I've given it a try but it really didn't do what I needed it to do.
Scintilla was a much better attempt, except it gave me problems with editor-synchronisation. The cause for this was a sort of incompatibility Iv'e got with the messaging system that Scintilla uses and the connection-system of Qt. I probably could have solved this (and I've did solved part of it), but it required me to make considerable changes in the Scintilla source code. I really didn't like the idea of maintaining a fork of Scintilla. (Btw. Scintilla is a very nice editor-component used by several products like Scite and Notepad++)
This lead me to think I could write an editor myself, so it would fit my needs. This shouldn't be that hard, just move the cursor and draw some letters (so I thought). Well I was very wrong :)
I really like Sublime Text, it really has really some awesome features, so while writing this editor I just implement one of my favorite features: multi-caret support.
Well this last decision really made the development a much harder, especially the undo part.
When the editor was at a certain level I needed support for syntax highlighting, I've first tried to implement a system like the one that Scintilla uses, because of the simplicity of implementing it. But after realizing that I needed to write a lexer for every language I really freaked out and decided to stick with the Textmate/Sublime grammar files.
But using textmate grammer-files required the inclusion of scopes to identify items in the text. So I build in supporting classes for scopes. To recognize these scopes I needed to run the regular expressions used in the grammar files.
Running these regexps in Qt with QRegExp doesn't work. Really it doesn't I tried it..!! I've included Oniguruma for this (The regexp engine of Textmate, Sublime and Ruby)
Now I was able to identify the scopes in the editor. When I had the scopes It was more then logical to use the theme files of Textmate because these were scope-based.
Why Opensource it?
Well I don't see any real big market for it. To be honestly I don't think the quality of the editor at this moment is at the required level for a commercial product. Also I hope to improve the quality of this product by open sourcing it. Though I'm a bit afraid for the criticism I might get on the written code, I hope that critics and remarks will improve my Qt coding knowledge and the overall quality of the editor component.
Also I really would like to see multi-caret support in many more products!
The Editor App
I've written an editor application for this text-component. The initial purpose was to create a simple application that could be used for playing with the component in a simple setting. The first version was a simple form of irresponsible code to quickly play with the thing. It still has a lot of dirty hacks in it.
The plan I have with this editor is to expand it to a full cross platform scriptable editor-environment.
I've build some binaries and placed the at https://bintray.com/gamecreature/edbee/edbee-app. Be warned though, I still need to figure out all dependencies of these executables (especially the windows version) and need a better way to distribute the editor.
There's still a lot of work todo..
If anybody is interested in helping please drop me an email ( rick, blommersit, nl )* or a tweet!@gamecreature :)
* Replace the comma/spaces with the correct two symbols